Middleware and AutoMapper in ASP.NET Core: A Developer's Guide
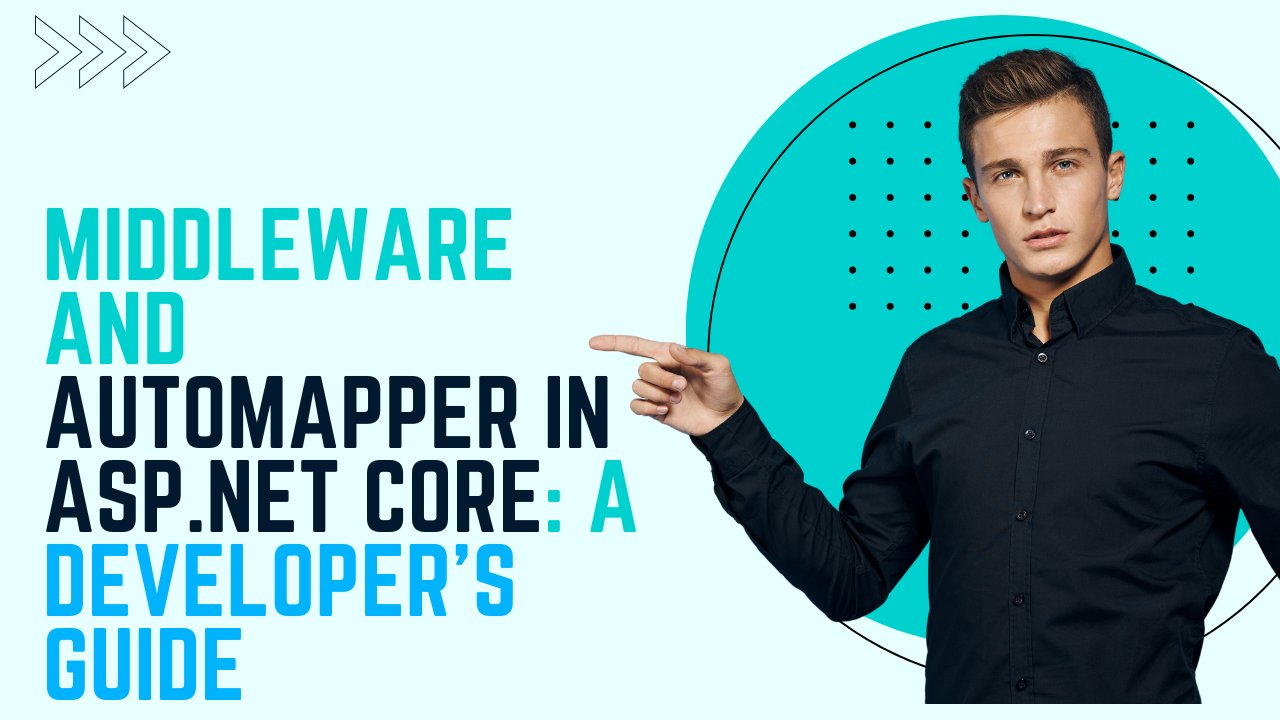
In the dynamic world of web development, ASP.NET Core stands out as a powerful and versatile framework. Among its many features, ASP.NET Core middleware and AutoMapper in .NET Core play crucial roles in creating efficient, maintainable, and scalable applications. Whether you're new to ASP.NET Core or a seasoned developer looking to deepen your knowledge, this guide will walk you through these essential components, helping you harness their full potential.
If you're eager to dive deeper into how middleware and AutoMapper can elevate your development process, consider exploring the asp.net core middleware tutorial. For those looking to streamline object mapping, the automapper in .net core guide offers comprehensive insights.
Understanding Middleware in ASP.NET Core
What is Middleware?
In ASP.NET Core, middleware is a key concept that refers to software components that are assembled into an application pipeline to handle requests and responses. Each piece of middleware in the pipeline has the opportunity to process incoming requests, handle them, or pass them to the next component in the chain. The ability to build a custom pipeline using ASP.NET Core middleware allows developers to create highly modular and maintainable applications.
The Role of Middleware in ASP.NET Core
Middleware components are executed in the order they are added to the pipeline, making their sequence crucial. Common tasks handled by middleware include authentication, logging, error handling, and request routing.
For example, a typical middleware pipeline might look like this:
public void Configure(IApplicationBuilder app)
{
app.UseAuthentication();
app.UseAuthorization();
app.UseStaticFiles();
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
In this example, ASP.NET Core middleware components are layered to first handle authentication and authorization before serving static files, routing requests, and mapping endpoints.
Creating Custom Middleware
One of the strengths of ASP.NET Core middleware is its extensibility. Developers can easily create custom middleware to handle specific application needs. Here's a basic example of custom middleware:
public class RequestLoggingMiddleware
{
private readonly RequestDelegate _next;
public RequestLoggingMiddleware(RequestDelegate next)
{
_next = next;
}
public async Task InvokeAsync(HttpContext context)
{
Console.WriteLine($"Request: {context.Request.Method} {context.Request.Path}");
await _next(context);
}
}
public void Configure(IApplicationBuilder app)
{
app.UseMiddleware<RequestLoggingMiddleware>();
}
This custom middleware logs every incoming request, showing how flexible and powerful ASP.NET Core middleware can be.
Introduction to AutoMapper in .NET Core
What is AutoMapper?
AutoMapper is a popular object-to-object mapping library that helps developers easily map properties from one object to another. In complex applications, it's common to work with different data models for various layers (e.g., domain models, view models, DTOs). Manually mapping properties between these models can be tedious and error-prone. AutoMapper in .NET Core simplifies this process by automatically mapping matching properties, reducing boilerplate code, and improving maintainability.
Setting Up AutoMapper in .NET Core
To get started with AutoMapper in .NET Core, you'll need to install the AutoMapper NuGet package. Once installed, you can configure AutoMapper in the Startup.cs file.
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddAutoMapper(typeof(Startup));
}
Next, create a mapping profile where you define the mappings between your source and destination objects:
public class MappingProfile : Profile
{
public MappingProfile()
{
CreateMap<SourceModel, DestinationModel>();
}
}
With this setup, AutoMapper will automatically map properties from SourceModel to DestinationModel whenever you use the IMapper interface.
Using AutoMapper in Your Application
Using AutoMapper in .NET Core is straightforward. You inject the IMapper interface into your controllers or services and use it to perform the mapping.
public class ExampleController : ControllerBase
{
private readonly IMapper _mapper;
public ExampleController(IMapper mapper)
{
_mapper = mapper;
}
public IActionResult Get()
{
var source = new SourceModel { Name = "John", Age = 30 };
var destination = _mapper.Map<DestinationModel>(source);
return Ok(destination);
}
}
In this example, the source object is automatically mapped to the destination object, with matching properties transferred seamlessly.
For a more in-depth understanding of how to implement and use AutoMapper, check out the automapper in .net core tutorial.
Middleware and AutoMapper: A Powerful Combination
Integrating AutoMapper with Middleware
In complex applications, there may be scenarios where you need to perform object mapping at the middleware level. For instance, you might have middleware that transforms an incoming request body into a specific model before passing it down the pipeline. Integrating ASP.NET Core middleware with AutoMapper in .NET Core allows you to handle these scenarios efficiently.
Here's an example of middleware that uses AutoMapper to map an incoming JSON request body to a specific model:
public class MappingMiddleware
{
private readonly RequestDelegate _next;
private readonly IMapper _mapper;
public MappingMiddleware(RequestDelegate next, IMapper mapper)
{
_next = next;
_mapper = mapper;
}
public async Task InvokeAsync(HttpContext context)
{
if (context.Request.Method == HttpMethods.Post)
{
var json = await new StreamReader(context.Request.Body).ReadToEndAsync();
var sourceObject = JsonConvert.DeserializeObject<SourceModel>(json);
var destinationObject = _mapper.Map<DestinationModel>(sourceObject);
context.Items["MappedObject"] = destinationObject;
}
await _next(context);
}
}
public void Configure(IApplicationBuilder app)
{
app.UseMiddleware<MappingMiddleware>();
}
In this scenario, the middleware maps the incoming request body to a DestinationModel object, which can then be accessed by other middleware or controllers down the pipeline.
Best Practices for Middleware and AutoMapper
- Keep Middleware Lightweight: While middleware can be powerful, it’s best to keep each middleware component focused on a specific task. Overloading middleware with too many responsibilities can lead to performance issues and code that's difficult to maintain.
- Use Profiles for AutoMapper: When configuring AutoMapper, it's a good practice to use profiles to organize your mappings. This keeps your mapping configuration clean and manageable.
- Test Middleware Independently: Ensure each piece of middleware is thoroughly tested independently before integrating it into your application pipeline. This helps in isolating issues and ensures that each component behaves as expected.
Advanced Middleware Techniques
Middleware Dependency Injection
One of the advanced features of ASP.NET Core middleware is its support for dependency injection. This allows middleware components to use services defined in the Startup.cs file, making them more flexible and easier to test.
public class LoggingMiddleware
{
private readonly RequestDelegate _next;
private readonly ILogger<LoggingMiddleware> _logger;
public LoggingMiddleware(RequestDelegate next, ILogger<LoggingMiddleware> logger)
{
_next = next;
_logger = logger;
}
public async Task InvokeAsync(HttpContext context)
{
_logger.LogInformation("Handling request: " + context.Request.Path);
await _next(context);
_logger.LogInformation("Finished handling request.");
}
}
In this example, the LoggingMiddleware uses dependency injection to access the ILogger service, making it easier to log information during the request lifecycle.
Ordering Middleware
The order in which you add middleware to the pipeline is crucial. Middleware components are executed in the order they're added, and reversing the order can lead to different behavior. It's important to carefully plan the sequence of your ASP.NET Core middleware to ensure that each component functions correctly.
Conclusion
Mastering ASP.NET Core middleware and AutoMapper in .NET Core is essential for developers aiming to build efficient, maintainable, and scalable web applications. Middleware provides a flexible way to handle requests and responses, while AutoMapper simplifies object mapping, reducing boilerplate code and improving maintainability. By understanding and effectively using these tools, you can take your ASP.NET Core development skills to the next level.
For further insights, explore the detailed guides on asp.net core middleware and automapper in .net core to enhance your development process.
FAQs
Q1: Can I use AutoMapper for complex object mappings?
Yes, AutoMapper can handle complex object mappings, including nested objects and collections. You can configure custom mappings and use resolvers to handle specific mapping scenarios.
Q2: How can I test custom middleware in ASP.NET Core?
You can test custom middleware by creating a test host using TestServer in your unit tests. This allows you to simulate HTTP requests and verify that your middleware